Google App Engine is a cloud computing platform for developing and hosting web applications in Google-managed data centers.This applications are sandbox and run across multiple servers
App Engine offers automatic scaling for web applications—as the number of requests increases for an application, App Engine automatically allocates more resources for the web application to handle the additional demand.
Google App Engine is free up to a certain level of consumed resources. Fees are charged for additional storage, bandwidth, or instance hours required by the application.It was first released as a preview version in April 2008, and came out of preview in September 2011.
Currently, the supported programming languages are Python, Java (and, by extension, other JVM languages such as Groovy, JRuby, Scala, Clojure), Go, and PHP. Go and PHP are in experimental status.[4] Google has said that it plans to support more languages in the future, and that the Google App Engine has been written to be language independent.
1.3 AppEngine technologies
App Engine uses the Jetty servlet container to host applications and supports the Java Servlet API in version 2.4. It provides access to databases via Java Data Objects (JDO) and the Java Persistence API (JPA). In the background App Engine uses Google Bigtable as the distributed storage system for persisting application data.
Google provides Memcache as a caching mechanism. Developers who want to code against the standard Java API can use the JCache implementation (based on JSR 107).
}
The creation of the EntityManagerFactory is time-consuming therefore we buffer it.
The following class provides the possibility to query for all todos and to add and delete todos.
App Engine offers automatic scaling for web applications—as the number of requests increases for an application, App Engine automatically allocates more resources for the web application to handle the additional demand.
Google App Engine is free up to a certain level of consumed resources. Fees are charged for additional storage, bandwidth, or instance hours required by the application.It was first released as a preview version in April 2008, and came out of preview in September 2011.
Currently, the supported programming languages are Python, Java (and, by extension, other JVM languages such as Groovy, JRuby, Scala, Clojure), Go, and PHP. Go and PHP are in experimental status.[4] Google has said that it plans to support more languages in the future, and that the Google App Engine has been written to be language independent.
Google App Engine for Java (GAE)
Google offers a cloud
computing infrastructure called
Google App Engine
(App Engine) for creating and
running
web applications.
App Engine
allows the dynamic allocation of system
resources for an
application
based on the
actual demand.
Currently
App Engine
supports
Python
and Java based applications. This includes
Java
Virtual Machine
(JVM)
based
languages, e.g.
Groovy
or
Scala. This article will cover the App Engine for Java (GAE/J)).
The App Engine offers frequently standard Java API's and
App
Engine
specific API's for the same task. If you want to be able to
port your
application
from
the AppEngine to other webcontainers, e.g.
Tomcat or
Jetty, you should only use
Java standard API.
1.3 AppEngine technologies
App Engine uses the Jetty servlet container to host applications and supports the Java Servlet API in version 2.4. It provides access to databases via Java Data Objects (JDO) and the Java Persistence API (JPA). In the background App Engine uses Google Bigtable as the distributed storage system for persisting application data.
Google provides Memcache as a caching mechanism. Developers who want to code against the standard Java API can use the JCache implementation (based on JSR 107).
The App Engine provides several services. For example the
Blobstore allows to upload and store and serve large data objects
(blobs) with a limit of 2 Gigabyte. To create a blob you
upload a file via an HTTP request.
Google App Engine supports the creation of several version of
your application. In the Admin Console you can select which version
should be active. Your active application "your-name" will be
accessible via the URL "http://your-name.appspot.com". Each version
can also
be accessed for example to test a new version. The version
are
accessable via "http://versionnumber.latest.your-name.appspot.com"
where version is for example "2" and "latest" is a fixed string.
App Engine runs a version of Java 6 but does not provide all
Java
classes, for example
Swing
and most AWT classes
are not
supported.
You cannot use Threads or frameworks which uses Threads. You can also not write to the filesystem and only read files which are part of your application. Certain "java.lang.System" actions, e.g. gc() or exit() will do nothing. You can not call JNI code. Reflection is possible for your own classes and standard Java classes but your cannot use reflection to access other classes outside your application.
See Java Whitelist for a full list of supported classes.
A servlet needs also to reply within 30 seconds otherwise a "com.google.apphosting.api.DeadlineExceededException" is thrown.
You cannot use Threads or frameworks which uses Threads. You can also not write to the filesystem and only read files which are part of your application. Certain "java.lang.System" actions, e.g. gc() or exit() will do nothing. You can not call JNI code. Reflection is possible for your own classes and standard Java classes but your cannot use reflection to access other classes outside your application.
See Java Whitelist for a full list of supported classes.
A servlet needs also to reply within 30 seconds otherwise a "com.google.apphosting.api.DeadlineExceededException" is thrown.
Google offers free hosting for websites which are not highly
frequented, e.g. 5 Millions page views. The price model for the
websites that exceed thier daily quota is listed on the
Google billing
documentation pages. The usage quotas
of the App Engine are constantly changing but, at
the time of this
writing, are around 5 millions pages views per month,
which translates
approx. in 6.5 CPU hours and 1 gigabyte of outbound
traffic.
Currently a user can create a maximum of 10 applications on the Google App Engine. The user can delete existing application in the Admin Console under Application Settings.
Currently a user can create a maximum of 10 applications on the Google App Engine. The user can delete existing application in the Admin Console under Application Settings.
Google offers a
Eclipse
plug-in that provides both
Google App Engine
and
GWT
development
capabilities.
Install the plugins from
http://dl.google.com/eclipse/plugin/3.7
via the
Eclipse update manager.
The installation will also setup the GWT and App Engine SDK into your Eclipse preferences. To check this use Window -> Preferences -> Google -> App Engine / Web Toolkit. The SDK are delivered as plugins is included in your Eclipse installation directory under "/plugins/".
The installation will also setup the GWT and App Engine SDK into your Eclipse preferences. To check this use Window -> Preferences -> Google -> App Engine / Web Toolkit. The SDK are delivered as plugins is included in your Eclipse installation directory under "/plugins/".
To deploy your application to the Google cloud you need a AppEngine
account. To get such an account you need a Google email account.
Open
the
URL
http://appengine.google.com/
and login with your Google account information. You
need to verify your
account via a valid phone number. After
providing
your phone number,
Google will text you a verification code
via SMS.
You will then type the
verification code into the verification
box
online.
The process of creating an application on the Google App Engine
involves the creation of an application on the Google App Engine
website. Afterwards you can locally create a web application and
upload this application to the created application on the Google App
Engine.
To create an application on the App Engine press the button "Create an application" and select an application name. You have to choose one which is still available. Remember this name because we will later use this in the creation of our demo application.
To create an application on the App Engine press the button "Create an application" and select an application name. You have to choose one which is still available. Remember this name because we will later use this in the creation of our demo application.
The Eclipse Plugin allows to run applications for the Google
App
Engine locally in an environment which simulates the environment
on
the App Engine. You also have a local admin console which allow
you to
see your local datastore, the task queue, inbound email and
XMPP
traffic. You find this local admin console on
"http://localhost:8888/_ah/admin/".
GAE/J supports
JPA (Java Persistence API)
1.0 for persisting data. As all data on the GAE/J are
stored in
Bigtable
some features of JPA are not supported. See
JPA on GAE/J
for details.
Alternatively to JPA you can also use JDO or the low-level API of the App Engine. The
datastorage facility is described in
Datastore Java API Overview.
The following will create a small "Todo" application using servlets
and
JSP's. The persistence will be handled by
JPA. For
an introduction into servlet and JSP programming please
see
Servlet and JSP development - Tutorial

Create a new project "de.vogella.gae.java.todo" via File > New
> Web Application Project. Package
name is "de.vogella.gae.java.todo".
The created project can already run. Right-click on your application, select run as-> Web Application
This should start Jetty on http://localhost:8888/ . Open the url in the browser and you should the possibility to select your servlet and to start it. If you select it you should see a "Hello, world" message.

The created project can already run. Right-click on your application, select run as-> Web Application

This should start Jetty on http://localhost:8888/ . Open the url in the browser and you should the possibility to select your servlet and to start it. If you select it you should see a "Hello, world" message.

Create the following class which will be our model. The
JPA
metadata is already added.
package de.vogella.gae.java.todo.model; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id;/** * Model class which will store the Todo Items * * @author Lars Vogel * */@Entity public class Todo { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; private String author; private String summary; private String description; private String url; boolean finished; public Todo(String author, String summary, String description, String url) { this.author = author; this.summary = summary; this.description = description; this.url = url; finished = false; } public Long getId() { return id; } public String getAuthor() { return author; } public void setAuthor(String author) { this.author = author; } public String getShortDescription() { return summary; } public void setShortDescription(String shortDescription) { this.s
6.4. Setup JPA and your DAO
To use JPA in your project you must create the JPA configuration file "persistence.xml" in the "META-INF" directory. This file contains the configuration that tells JPA to use the App Engine datastore.<?xml version="1.0" encoding="UTF-8" ?> <persistence xmlns="http://java.sun.com/xml/ns/persistence" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/persistence http://java.sun.com/xml/ns/persistence/persistence_1_0.xsd" version="1.0"> <persistence-unit name="transactions-optional"> <provider>org.datanucleus.store.appengine.jpa.DatastorePersistenceProvider</provider> <properties> <property name="datanucleus.NontransactionalRead" value="true"/> <property name="datanucleus.NontransactionalWrite" value="true"/> <property name="datanucleus.ConnectionURL" value="appengine"/> </properties> </persistence-unit> </persistence>
The creation of the EntityManagerFactory is time-consuming therefore we buffer it.
package de.vogella.gae.java.todo.dao; import javax.persistence.EntityManagerFactory; import javax.persistence.Persistence; public class EMFService { private static final EntityManagerFactory emfInstance = Persistence .createEntityManagerFactory("transactions-optional"); private EMFService() { } public static EntityManagerFactory get() { return emfInstance; } }
The following class provides the possibility to query for all todos and to add and delete todos.
package de.vogella.gae.java.todo.dao; import java.util.List; import javax.persistence.EntityManager; import javax.persistence.Query; import de.vogella.gae.java.todo.model.Todo; public enum Dao { INSTANCE; public List<Todo> listTodos() { EntityManager em = EMFService.get().createEntityManager(); // read the existing entries Query q = em.createQuery("select m from Todo m"); List<Todo> todos = q.getResultList(); return todos; } public void add(String userId, String summary, String description, String url) { synchronized (this) { EntityManager em = EMFService.get().createEntityManager(); Todo todo = new Todo(userId, summary, description, url); em.persist(todo); em.close(); } } public List<Todo> getTodos(String userId) { EntityManager em = EMFService.get().createEntityManager(); Query q = em .createQuery("select t from Todo t where t.author = :userId"); q.setParameter("userId", userId); List<Todo> todos = q.getResultList(); return todos; } public void remove(long id) { EntityManager em = EMFService.get().createEntityManager(); try { Todo todo = em.find(Todo.class, id); em.remove(todo); } finally { em.close(); } } }
Create the following two servlets. The first will be called if
a new Todo is created the second one if a Todo is finished.
package de.vogella.gae.java.todo; import java.io.IOException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import com.google.appengine.api.users.User; import com.google.appengine.api.users.UserService; import com.google.appengine.api.users.UserServiceFactory; import de.vogella.gae.java.todo.dao.Dao; @SuppressWarnings("serial") public class ServletCreateTodo extends HttpServlet { public void doPost(HttpServletRequest req, HttpServletResponse resp) throws IOException { System.out.println("Creating new todo "); User user = (User) req.getAttribute("user"); if (user == null) { UserService userService = UserServiceFactory.getUserService(); user = userService.getCurrentUser(); } String summary = checkNull(req.getParameter("summary")); String longDescription = checkNull(req.getParameter("description")); String url = checkNull(req.getParameter("url")); Dao.INSTANCE.add(user.getUserId(), summary, longDescription, url); resp.sendRedirect("/TodoApplication.jsp"); } private String checkNull(String s) { if (s == null) { return ""; } return s; } }
package de.vogella.gae.java.todo; import java.io.IOException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import de.vogella.gae.java.todo.dao.Dao; public class ServletRemoveTodo extends HttpServlet { private static final long serialVersionUID = 1L; public void doGet(HttpServletRequest req, HttpServletResponse resp) throws IOException { String id = req.getParameter("id"); Dao.INSTANCE.remove(Long.parseLong(id)); resp.sendRedirect("/TodoApplication.jsp"); } }
In the folder "war" create the following JSP
"TodoApplication.jsp".
The JSP refers to a css file. Create a folder "war/css" and create the following file "main.css".
<%@ page contentType="text/html;charset=UTF-8" language="java" %> <%@ page import="java.util.List" %> <%@ page import="com.google.appengine.api.users.User" %> <%@ page import="com.google.appengine.api.users.UserService" %> <%@ page import="com.google.appengine.api.users.UserServiceFactory" %> <%@ page import="de.vogella.gae.java.todo.model.Todo" %> <%@ page import="de.vogella.gae.java.todo.dao.Dao" %> <!DOCTYPE html> <%@page import="java.util.ArrayList"%> <html> <head> <title>Todos</title> <link rel="stylesheet" type="text/css" href="css/main.css"/> <meta charset="utf-8"> </head> <body> <% Dao dao = Dao.INSTANCE; UserService userService = UserServiceFactory.getUserService(); User user = userService.getCurrentUser(); String url = userService.createLoginURL(request.getRequestURI()); String urlLinktext = "Login"; List<Todo> todos = new ArrayList<Todo>(); if (user != null){ url = userService.createLogoutURL(request.getRequestURI()); urlLinktext = "Logout"; todos = dao.getTodos(user.getUserId()); } %> <div style="width: 100%;"> <div class="line"></div> <div class="topLine"> <div style="float: left;"><img src="images/todo.png" /></div> <div style="float: left;" class="headline">Todos</div> <div style="float: right;"><a href="<%=url%>"><%=urlLinktext%></a> <%=(user==null? "" : user.getNickname())%></div> </div> </div> <div style="clear: both;"/> You have a total number of <%= todos.size() %> Todos. <table> <tr> <th>Short description </th> <th>Long Description</th> <th>URL</th> <th>Done</th> </tr> <% for (Todo todo : todos) {%> <tr> <td> <%=todo.getShortDescription()%> </td> <td> <%=todo.getLongDescription()%> </td> <td> <%=todo.getUrl()%> </td> <td> <a class="done" href="/done?id=<%=todo.getId()%>" >Done</a> </td> </tr> <%} %> </table> <hr /> <div class="main"> <div class="headline">New todo</div> <% if (user != null){ %> <form action="/new" method="post" accept-charset="utf-8"> <table> <tr> <td><label for="summary">Summary</label></td> <td><input type="text" name="summary" id="summary" size="65"/></td> </tr> <tr> <td valign="description"><label for="description">Description</label></td> <td><textarea rows="4" cols="50" name="description" id="description"></textarea></td> </tr> <tr> <td valign="top"><label for="url">URL</label></td> <td><input type="url" name="url" id="url" size="65" /></td> </tr> <tr> <td colspan="2" align="right"><input type="submit" value="Create"/></td> </tr> </table> </form> <% }else{ %> Please login with your Google account <% } %> </div> </body> </html>
The JSP refers to a css file. Create a folder "war/css" and create the following file "main.css".
body { margin: 5px; font-family: Arial, Helvetica, sans-serif; } hr { border: 0; background-color: #DDDDDD; height: 1px; margin: 5px; } table th { background:#EFEFEF none repeat scroll 0 0; border-top:1px solid #CCCCCC; font-size:small; padding-left:5px; padding-right:4px; padding-top:4px; vertical-align:top; text-align:left; } table tr { background-color: #e5ecf9; font-size:small; } .topLine { height: 1.25em; background-color: #e5ecf9; padding-left: 4px; padding-right: 4px; padding-bottom: 3px; padding-top: 2px; } .headline { font-weight: bold; color: #3366cc; } .done { font-size: x-small; vertical-align: top; } .email { font-size: x-small; vertical-align: top; } form td { font-size: smaller; }
Change
web.xml
in folder /war/WEB-INF" to the following. It
will create the right servlet mapping and will set the JSP as start
page.
<?xml version="1.0" encoding="utf-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" version="2.5"> <servlet> <servlet-name>CreateNewTodo</servlet-name> <servlet-class>de.vogella.gae.java.todo.ServletCreateTodo</servlet-class> </servlet> <servlet> <servlet-name>RemoveTodo</servlet-name> <servlet-class>de.vogella.gae.java.todo.ServletRemoveTodo</servlet-class> </servlet> <servlet-mapping> <servlet-name>RemoveTodo</servlet-name> <url-pattern>/done</url-pattern> </servlet-mapping> <servlet-mapping> <servlet-name>CreateNewTodo</servlet-name> <url-pattern>/new</url-pattern> </servlet-mapping> <welcome-file-list> <welcome-file>TodoApplication.jsp</welcome-file> </welcome-file-list> </web-app>
You should be able to run your application locally, login and
create and
delete "Todos".

To deploy your application to the GAE you need to maintain your
application ID in the file "web/WEB-INF/appengine-web.xml". In the
tag "application" maintain the application you have created in
GAE/J Registration. In my example I use the application "vogellatodo".
<?xml version="1.0" encoding="utf-8"?> <appengine-web-app xmlns="http://appengine.google.com/ns/1.0"> <application>vogellatodo</application> <version>1</version> <!-- Configure java.util.logging --> <system-properties> <property name="java.util.logging.config.file" value="WEB-INF/logging.properties"/> </system-properties> </appengine-web-app>
Now deploy your application to the Google cloud. The following
assumes that you have already created your account.
Right-click on your project and select Google > App Engine Settings... from the context menu. Check that the application ID is as you maintained it in "appengine-web.xml".
Right-click on your project, Select Google -> Deploy to App Engine. You need to login with your Google account information.
After the upload completes you will find your application online under http://application-id.appspot.com, e.g. in my case http://vogellatodo.appspot.com.
Right-click on your project and select Google > App Engine Settings... from the context menu. Check that the application ID is as you maintained it in "appengine-web.xml".

Right-click on your project, Select Google -> Deploy to App Engine. You need to login with your Google account information.

After the upload completes you will find your application online under http://application-id.appspot.com, e.g. in my case http://vogellatodo.appspot.com.
AppEngine allows your application to send and receive emails. We
will use the receive email feature
to be able to receive new TODOs via
email.
To receive emails in your application you have to declare that your application is allowed to use the mail service. Add the service "mail" to your "appengine-web.xml".
After redeploying your app you can check in the admin console of the appengine under Application Settings if incoming email is allowed.
After defining that your application can receive email you need to define the email address for your application. The email address would be:
This address follows the pattern: "user@domain". The domainis based on the applicationid, e.g. "applicationid.appspotmail.com". Please note that string@appid.appspotmail.com appspotmail.com is different from appspot.com , which we use in the domain name of our application. You can add use any user you want as long as you provide a mapping to a servlet in
Via the
We will define that one servlet receives the email for all users. Add the following servlet mappings to your
Create the following servlet which will process your email.
To test your email functionality start your application and open the admin console on "http://localhost:8888/_ah/admin/". Select Inbound Mail and maintain some test data. Make sure the To email address match the servlet mapping for the email address.
Deploy your application. Send an email to "whatever@applicationid.appspotmail.com". Login to your application. You should get a new Todo with the summary of the email header and the description of the email body.
To receive emails in your application you have to declare that your application is allowed to use the mail service. Add the service "mail" to your "appengine-web.xml".
<?xml version="1.0" encoding="utf-8"?> <appengine-web-app xmlns="http://appengine.google.com/ns/1.0"> <application>vogellatodo</application> <version>1</version> <!-- Configure java.util.logging --> <system-properties> <property name="java.util.logging.config.file" value="WEB-INF/logging.properties"/> </system-properties> <inbound-services> <service>mail</service> </inbound-services> </appengine-web-app>
After redeploying your app you can check in the admin console of the appengine under Application Settings if incoming email is allowed.

After defining that your application can receive email you need to define the email address for your application. The email address would be:
user@appid.appspotmail.com
This address follows the pattern: "user@domain". The domainis based on the applicationid, e.g. "applicationid.appspotmail.com". Please note that string@appid.appspotmail.com appspotmail.com is different from appspot.com , which we use in the domain name of our application. You can add use any user you want as long as you provide a mapping to a servlet in
web.xml
.
Via the
web.xml
you define which servlet
answers to which user.
The url
pattern must start with "/_ah/mail/" to
tell AppEngine that this
is a
mapping for receiving emails. The url
pattern can include
wildcards.
We will define that one servlet receives the email for all users. Add the following servlet mappings to your
web.xml
, make sure you
replace the string
"applicationid" with your real id. "
<?xml version="1.0" encoding="utf-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" version="2.5"> <servlet> <servlet-name>CreateNewTodo</servlet-name> <servlet-class>de.vogella.gae.java.todo.ServletCreateTodo</servlet-class> </servlet> <servlet> <servlet-name>RemoveTodo</servlet-name> <servlet-class>de.vogella.gae.java.todo.ServletRemoveTodo</servlet-class> </servlet> <servlet-mapping> <servlet-name>RemoveTodo</servlet-name> <url-pattern>/done</url-pattern> </servlet-mapping> <servlet-mapping> <servlet-name>CreateNewTodo</servlet-name> <url-pattern>/new</url-pattern> </servlet-mapping> <servlet> <servlet-name>Email</servlet-name> <servlet-class>de.vogella.gae.java.todo.EmailServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>Email</servlet-name> <url-pattern>/_ah/mail/*</url-pattern> </servlet-mapping> <welcome-file-list> <welcome-file>TodoApplication.jsp</welcome-file> </welcome-file-list> </web-app>
Create the following servlet which will process your email.
package de.vogella.gae.java.todo; import java.io.IOException; import java.util.Properties; import javax.mail.Address; import javax.mail.MessagingException; import javax.mail.Multipart; import javax.mail.Part; import javax.mail.Session; import javax.mail.internet.MimeMessage; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import org.datanucleus.sco.simple.GregorianCalendar; import com.google.appengine.api.users.User; import de.vogella.gae.java.todo.dao.Dao; public class EmailServlet extends HttpServlet { private static final long serialVersionUID = 1L; public void doPost(HttpServletRequest req, HttpServletResponse resp) throws IOException { Properties props = new Properties(); Session email = Session.getDefaultInstance(props, null); try { MimeMessage message = new MimeMessage(email, req.getInputStream()); String summary = message.getSubject(); String description = getText(message); Address[] addresses = message.getFrom(); User user = new User(addresses[0].toString(), "gmail.com"); Dao.INSTANCE.add(user.getNickname(), summary, description, "", GregorianCalendar.getInstance()); } catch (Exception e) { e.printStackTrace(); } } private boolean textIsHtml = false;/** * Return the primary text content of the message. */private String getText(Part p) throws MessagingException, IOException { if (p.isMimeType("text/*")) { String s = (String)p.getContent(); textIsHtml = p.isMimeType("text/html"); return s; } if (p.isMimeType("multipart/alternative")) { // prefer html text over plain text Multipart mp = (Multipart)p.getContent(); String text = null; for (int i = 0; i < mp.getCount(); i++) { Part bp = mp.getBodyPart(i); if (bp.isMimeType("text/plain")) { if (text == null) text = getText(bp); continue; } else if (bp.isMimeType("text/html")) { String s = getText(bp); if (s != null) return s; } else { return getText(bp); } } return text; } else if (p.isMimeType("multipart/*")) { Multipart mp = (Multipart)p.getContent(); for (int i = 0; i < mp.getCount(); i++) { String s = getText(mp.getBodyPart(i)); if (s != null) return s; } } return null; } }
To test your email functionality start your application and open the admin console on "http://localhost:8888/_ah/admin/". Select Inbound Mail and maintain some test data. Make sure the To email address match the servlet mapping for the email address.
Deploy your application. Send an email to "whatever@applicationid.appspotmail.com". Login to your application. You should get a new Todo with the summary of the email header and the description of the email body.
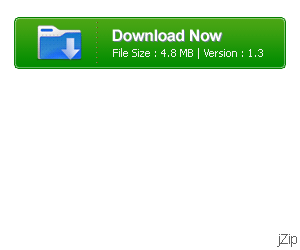
You can define cron jobs in your application. Via a cron job on
th AppEngine you
can access a URL according to a time pattern which is
defined similar to the cron jobs on Unix. You define your cron jobs in
a file "cron.xml" in the
This cron job will call the local website /count every minute.
You can check you cron jobs in the admin console.
WEB-INF
folder of you application.
<?xml version="1.0" encoding="UTF-8"?> <cronentries> <cron> <url>/count</url> <description>Calls the webpage /count every minute</description> <schedule>every 1 minutes</schedule> </cron> </cronentries>
This cron job will call the local website /count every minute.
You can check you cron jobs in the admin console.

Tip
Please note that you should avoid running unnecessary cron jobs as this increases the load of the AppEngine.
You can use
standard Java Logging
for logging on
the
App Engine. For example to define a logger in your
class EmailServlet use the following.
To log a message use the following.
You find your logs on the Admin Console. the For further information see Java Logging Tutorial and Logging on the GAE/J.
private static final Logger log = Logger.getLogger(EmailServlet.class.getName());
To log a message use the following.
log.info("Your information log message"); log.warning("Your warning log message"); log.severe("Your severe log message");
You find your logs on the Admin Console. the For further information see Java Logging Tutorial and Logging on the GAE/J.
The Admin Console provides you with a lot of information about
your application. For example you can acces the data stored in the
datastore or look at the log files of your application.
You can also use standard Java Logging for creating custom logs on the GAE/J. See Java Logging Tutorial and Logging on the GAE/J.

You can also use standard Java Logging for creating custom logs on the GAE/J. See Java Logging Tutorial and Logging on the GAE/J.
You can also extend the admin console with own pages. Create
the following entry in "appengine-web.xml".
We will just use a static HTML for the example. Create the following file in the
If you redeploy your application you have another entry in your Admin console.
<admin-console> <page name="Static List" url="/static.html" /> </admin-console>
We will just use a static HTML for the example. Create the following file in the
WEB-INF
folder.
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <html> <head> <meta http-equiv="content-type" content="text/html; charset=UTF-8"> <title>Integrated into the Admin Console</title> </head> <body> <h1>Hello Admin Console!</h1> <table> <tr> <td colspan="2" style="font-weight:bold;">This could be a dynamic site:</td> </tr> <tr> <td>But it isn't.</td> </tr> </table> </body> </html>
If you redeploy your application you have another entry in your Admin console.

No comments:
Post a Comment